Accordion
The Accordion
component creates a set of interactive headings that hide and show content sections, with Keyboard Support
Demo
Add the multiple
property to the tonic-accordion
component to allow multiple sections to be expanded at one time.
Code
HTML
<tonic-accordion id="my-accordion">
<tonic-accordion-section
name="bucket-test-1"
id="bucket-test-1"
data="preview"
label="Accordion Test 1">
Whatever
</tonic-accordion-section>
<tonic-accordion-section
name="bucket-test-2"
id="bucket-test-2"
label="Accordion Test 2">
Some Content
</tonic-accordion-section>
<tonic-accordion-section
name="bucket-test-3"
id="bucket-test-3"
label="Accordion Test 3">
The visual design includes features intended to help users understand that the accordion provides enhanced keyboard navigation functions. When an accordion header button has keyboard focus, the styling of the accordion container and all its header buttons is changed.
</tonic-accordion-section>
</tonic-accordion>
Api
Properties
for TonicAccordion
Property | Type | Description | Default |
---|---|---|---|
multiple |
number | Allow multiple sections to be expanded at one time. | 0 |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
for TonicAccordionSection
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. |
|
name |
string | Adds the name attribute. |
|
label |
string | Pass into | 0 |
Instance Members
Property | Description |
---|---|
click() |
Click event |
Badge
The Badge
component creates a notification badge with a counter and unread alert.
Demo
Code
To update the notification count, pass in the count
property.
HTML
<tonic-badge id="my-badge"></tonic-badge>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. |
|
name |
string | Adds the name attribute. |
|
count |
string | Updates the count property. |
0 |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Members
Property | Description |
---|---|
value |
Getter/setter for the badge count. |
Button
A Button
component with a built-in loading state.
Demo
Code
HTML
<tonic-button
async="true"
id="tonic-button-example"
value="click-me">Click me
</tonic-button>
CSS
An example of how to modify a button with a specific class.
tonic-button.danger {
--tonic-button-text: rgba(255, 255, 255, 1);
--tonic-button-background: rgba(240, 102, 83, 1);
--tonic-button-background-hover: rgba(250, 112, 93, 1);
--tonic-button-background-focus: rgba(245, 106, 88, 1);
}
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Button with id attribute. |
|
name |
string | Button with a name attribute. |
|
type |
string | Type of button (i.e. submit). | |
value |
string | Value of the button. | Submit |
disabled |
boolean | Button with the disabled attribute. |
false |
autofocus |
boolean | Button with the autofocus attribute. |
false |
async |
boolean | Make button asynchronous. | false |
is-active |
boolean | Active button. | false |
width |
string | Width of the button. | 150px |
height |
string | Height of the button. | 38px |
radius |
string | Radius of the button. | 2px |
background-color |
string | Button background color. | |
text-color |
string | TextColor of the button. | |
href |
string | Add a location (url) for click event. | |
target |
string | If you specify target, it will open an href in a new window, unless target is _self |
_self |
tabindex |
number | Add a tabindex for the button. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
symbol-id |
string | When type=icon , this id will be used to refer to the svg symbol. |
|
sizse |
string | When type=icon , this id will be used as the size of the icon. |
Instance Methods & Members
Method | Description |
---|---|
click() |
Click event |
loading(state) |
Removes loading from an async button. |
Chart
An example component that uses chart.js 2.8.0
.
Demo
Code
HTML
Options is optional and contains any valid chartjs configuration options.
const opts = {
tooltips: {
enabled: false
},
legend: {
display: false
},
drawTicks: true,
drawBorder: true
}
<tonic-chart
type="horizontalBar"
width="300"
height="150px"
options="${opts}"
chart-library="${require('chart.js')}"
src="/chartdata.json">
</tonic-chart>
JSON
{
"labels": ["Foo", "Bar", "Bazz"],
"datasets": [{
"label": "Quxx (millions)",
"backgroundColor": ["#c3c3c3", "#f06653", "#8f8f8f"],
"data": [278, 467, 34]
}]
}
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
title |
string | The title of the chart. | |
type |
string | The type of the bar chart. | |
chart-library |
function | The require('chart.js') module |
|
src |
object or string | The data for the chart. A url or an object. | |
tooltip |
bool | Show or don't show the tooltip. | |
width |
string | Width of the chart (include the unit, % , px etc). |
|
height |
string | Height of the chart (include the unit, % , px , etc). |
Instance Methods & Members
Method | Description |
---|---|
draw(Object, Object) |
Draws (or re-draws) the chart. The first parameter is the data and the second is options. |
setChart(Function) |
Sets the chart library; call with setChart(require('chart.js)) . This is an alternative to the chart-library HTML attribute |
Checkbox
The Checkbox
component is used to create a styled checkbox, with or without a
label. You can also create a custom checkbox using SVG icons.
Note: This component requires the
tonic-sprite
component.
Demo
Code
HTML
<tonic-checkbox
id="tonic-checkbox-example"
label="Checkbox with Label">
</tonic-checkbox>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds id attribute. required |
|
name |
string | Adds name attribute. |
|
disabled |
boolean | Adds disabled attribute. |
false |
checked |
boolean | Adds checked attribute. |
false |
size |
string | Changes the width and height of the icon. |
18px |
color |
string | Changes the color of the icon. | --primary |
tabindex |
number | Add a tabindex for the checkbox. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
virtual |
boolean | Makes the checkbox non-clickable, you need to set the checked state manually | false |
Instance Properties
Method | Description |
---|---|
value |
A getter/setter that returns true if the checkbox is checked or false if the checkbox is not checked. |
Static Methods
Method | Description |
---|---|
addIcon(state, fn) |
Add a custom SVG as the icon for the given state Where the state parameter is either on or off and the fn parameter is a function that returns your SVG's xml. The function will receive the color prop as an argument. |
Dialog
All dialogs have different use cases. Anything could go inside them. So this class tries to accomodate a wide range of use cases by being the minimum of what is needed to start with.
Note: This component requires the
tonic-sprite
component.
Demo
Code
HTML
<button id="open-dialog">Open Dialog</button>
<tonic-dialog id="example-dialog">
<show-random>
</show-random>
</tonic-dialog>
JS
class ShowRandom extends Tonic {
async click (e) {
if (Tonic.match(e.target, '#update')) {
this.state.message = String(Math.random())
this.reRender()
}
}
render () {
return this.html`
<header>Dialog</header>
<main>
${this.state.message || 'Ready'}
</main>
<footer>
<tonic-button id="update">Update</tonic-button>
</footer>
`
}
}
Tonic.add(ShowRandom)
const link = document.getElementById('open-dialog')
const dialog = document.getElementById('example-dialog')
link.addEventListener('click', async e => dialog.show())
CSS
Example styles that a project might set up outside the component's css.
.tonic--dialog {
display: grid;
grid-template-rows: 44px 1fr 80px;
}
.tonic--dialog > header {
user-select: none;
line-height 44px;
text-align center;
color var(--tonic-medium);
display block;
}
.tonic--dialog > main {
padding 0 30px 10px;
position relative;
text-align center;
}
.tonic--dialog > footer {
user-select: none;
text-align center;
background-color var(--tonic-window);
padding 16px;
text-align center;
width 100%;
display block;
}
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds an id attribute. |
|
width |
string | Sets the width of the dialog. | |
height |
string | Sets the height of the dialog. |
Instance Methods & Members
Method | Description |
---|---|
show() |
Shows the dialog. Returns a promise that can be awaited while the animation finishes. |
hide() |
Hides the dialog. Returns a promise that can be awaited while the animation finishes. |
Form
The form
component implements input
and change
events that collect
the values of any child component that has the data-key
attribute. The values
colected are stored on the tonic-form
state and accessible from the value
property.
The value of data-key
expects a dot notation
to determine what the value
on the form will be.
Demo
Code
HTML
<tonic-form id="form-example">
<tonic-input data-key="ka" required="true" id="a">
</tonic-input>
<tonic-select
data-key="foo.kb"
id="b"
required="true"
>
<option value="" selected="true" disabled="true">Select One</option>
<option value="a">a</option>
<option value="b">b</option>
</tonic-select>
<tonic-input id="c" value="vc">
</tonic-input>
<tonic-input required="true" data-key="bar.0.buzz" id="d" value="vd">
</tonic-input>
<tonic-button disabled="true" id="form-submit">Submit</tonic-button>
</tonic-form>
JS
const form = document.getElementById('form-example')
const expected = {
ka: 'va',
foo: {
kb: 'vb'
},
bar: [{ buzz: 'vd' }]
}
assert(form.value === expected)
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Input with id attribute. required |
|
fill |
boolean | If specified, upon render, any child-element value that that specifies data-key will be filled with the form's coresponding state value unless a value is specified as a property. |
Instance Methods
Method | Description |
---|---|
validate({ decorate }) |
Reset and run all validation for all components that implement setValid() and setInvalid() . If decorate is set to false , this function only checks for validity. |
setValid() |
Attempt to call setValid() on all form elements that implement it. |
Instance Members
Property | Description |
---|---|
value |
Getter/setter for the value of the form. |
Icon
The Icon
component is used to create an SVG icon with a custom size and color.
Your single SVG sprite file should have the following base structure, using <symbol>
and an id
to refer to the specific icon:
<svg version="1.1" xmlns="http://www.w3.org/2000/svg">
<symbol id="example">
<path />
</symbol>
</svg>
Demo
Code
HTML
<tonic-icon
symbol-id="example"
src="/images/sprite.svg"
fill="red"
size="40px">
</tonic-icon>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
size |
string | Changes the width and height of the icon. | 25px |
fill |
string | Changes the color of the icon. | var(--tonic-primary) |
src |
string | Optional, a custom file to use as the the source. | ./sprite.svg |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Input
The Input
component creates an input that can be invalidated.
Demo
Code
HTML
<tonic-input
label="Email Address"
type="email"
width="280px"
id="tonic-input-example"
placeholder="Enter a valid email address"
spellcheck="false"
error-message="Invalid Email">
</tonic-input>
Native auto-complete is also supported.
<tonic-input
label="Email Address"
type="email"
id="tonic-input-example"
>
<option value="a">primary@foo.com</option>
<option value="b">secondary@foo.com</option>
</tonic-input>
The input will validate automatically if you specify the type
or the pattern
attribute.
You can also validate or invalidate an input with Javascript using the methods setValid()
and setInvalid()
JS
setInvalid.addEventListener('click', (e) => {
input.setInvalid('There was a problem')
})
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Input with id attribute. required |
|
aria-invalid |
boolean | Adds aria-invalid attribute. |
false |
aria-labelledby |
string | Adds aria-labelledby attribute. |
false |
disabled |
boolean | Makes the input disabled . |
false |
error-message |
string | Changes error message text. | |
invalid |
boolean | Adds the invalid attribute. |
false |
label |
string | Adds a label to the input. | |
name |
string | Input with name attribute. |
|
pattern |
string | Regex for checking value. | |
placeholder |
string | Inserts placeholder text. |
|
position |
string | The position of an icon, if specified. | |
radius |
string | Radius of the input. | 3px |
required |
boolean | Makes the input required . |
false |
readonly |
boolean | Makes the input readonly . |
false |
spellcheck |
boolean | Enable spellcheck on the input. |
false |
src |
string | The src to use for the icon. | |
symbol-id |
string | The symbol id to use for the icon. | |
fill |
string | The fill color to use for the icon. | |
color |
string | The path color to use for the icon. | |
tabindex |
number | Add a tabindex for the input. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
type |
string | Type of input (text, password, email). | text |
width |
string | Width of the input. | 250px |
Instance Methods
Method | Description |
---|---|
setValid() |
Sets the input to valid. |
setInvalid(msg) |
Invalidate the input. |
Instance Members
Property | Description |
---|---|
value |
Getter/setter for the value of the input. |
Loader
Renders an animated SVG-based loader. Placed inside a relative or absolute positioned container, it will center itself.
Demo
Code
HTML
<tonic-loader>
</tonic-loader>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
timeout |
number | How long to spin (-1 will be indefinite, though this is not recommended because animations can tax the CPU). |
Panel
Similar to dialog, just a wrapper with hide and show.
Note: This component requires the
tonic-sprite
component.
Demo
Code
HTML
<tonic-panel
id="content-panel-example"
overlay="true">
</tonic-panel>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. |
|
position |
string | Changes the position of the panel (left or right ). |
right |
width |
string | Width of the panel. | |
width-mobile |
string | Width of the panel on mobile. |
Instance Methods & Members
Method | Description |
---|---|
show() |
Shows the panel. |
hide() |
Hides the panel. |
Popover
The popover
component creates a popover activated by a click event. It is positioned in relation to the trigger.
Demo
Code
HTML
<tonic-popover id="popover-example" width="175px" for="popover-example-trigger">
<div>Item 1</div>
<div>Item 2</div>
<div>Item 3</div>
</tonic-popover>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
for |
string | Adds a for attribute. required |
|
width |
string | Changes the width style. |
auto |
height |
string | Changes height style. |
auto |
open |
boolean | Determines the default state of the popover. | false |
padding |
string | Changes padding style. |
15px |
margin |
number | Changes margin style. |
10 |
position |
string | Changes position of popover. Can be one of: top , topleft , topright , bottom , bottomleft , or bottomright . |
bottomleft |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods & Members
Method | Description |
---|---|
show() |
Shows the popover. |
hide() |
Hides the popover. |
ProfileImage
The ProfileImage
component is used to create an SVG icon with a custom size
and color.
Note: This component requires the
tonic-sprite
component.
Demo
Code
HTML
<tonic-profile-image
id="profile-image-example-editable"
size="150px"
editable="true">
</tonic-profile-image>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. |
|
name |
string | Adds the name attribute. |
|
src |
string | Add an image source. | |
size |
string | Changes the width and height of the image. | 25px |
radius |
string | Change the border-radius of the image. | 5px |
border |
string | Change the border of the image (i.e. '1px solid white'). | |
editable |
boolean | Add an edit overlay. | false |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Events
Name | Description |
---|---|
change |
Emitted when the src changes. |
error |
Emitted when there was a problem reading the provided input. |
ProgressBar
The ProgressBar
component creates an updatable progress bar.
Demo
Code
HTML
<tonic-progress-bar id="progress-bar-example">
</tonic-progress-bar>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
width |
string | Changes the width of the progress bar. | 280px |
height |
string | Changes the height of the progress bar. | 15px |
color |
string | Changes the color of the progress bar. | --accent |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods
Method | Description |
---|---|
setProgress(number) |
Sets the progress (percentage). |
Instance Members
Method | Description |
---|---|
value |
A getter/setter that provides the current percentage value of the progress bar. |
Range
The Range
component creates a range input, or slider.
Demo
Code
HTML
<tonic-range
label="The value is %i%"
id="tonic-range-example">
</tonic-range>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Input with id attribute. Required |
|
name |
string | Input with name attribute. |
|
disabled |
boolean | Makes the input disabled . |
false |
width |
string | Width of the track. | 250px |
height |
string | Height of the track. | 4px |
radius |
string | Radius of the track. | 3px |
min |
string | Least possible value. | 0 |
max |
string | Greatest possible value. | 100 |
step |
string | Number the value must adhere to. | 1 |
label |
string | Label that displays the current value. Use %i, %f, etc to represent the value. | false |
thumbColor |
string | Color of the slider "thumb" | var(--tonic-window) |
thumbRadius |
string | Radius of the slider "thumb" | 50px |
value |
string | Default value | 50 |
tabindex |
number | Add a tabindex for the range. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Members
Property | Description |
---|---|
value |
Get the current value of the range input. |
Relative-Time
The Relative-Time
component creates a relative text representation of a
date value. This is almost entirely based on Github's Time Elements.
Demo
Code
HTML
<tonic-relative-time
date="${new Date()}"
refresh="1e4"
locale="en"
</tonic-relative-time>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
locale |
string | Represents a Unicode locale identifier. | default |
date |
string, Date | A valid javascript date object or a parsable javascript date string. | new Date() |
Router
The Router
component will render its children components if the browser's current url matches its path
property. This component will detect pushstate
, popstate
and replacestate
events and re-render with the attributes of the url as props.
Demo
Code
HTML
<tonic-router id="page1" path="/examples.html">
<i>Hello, World</i>
</tonic-router>
<tonic-router id="page2" path="/bar/:number">
<b>number</b> prop has the value <b id="page2-number"></b>.
</tonic-router>
<tonic-router none>404</tonic-router>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
path |
string | A tokenized string to match against the current url, /books/:book for example. |
|
none |
string | If specified, and no matches have been made so far, this component will render. | |
id |
string | If specified, provides a way to reference the component instance and listen for events on it. |
Events
Name | Description |
---|---|
match |
Emitted when a content section receives the show class because it matches the current url. |
Select
The Select
component creates a select input.
Demo
Code
HTML
<tonic-select name="select">
<option value="a">Option A</option>
<option value="b">Option B</option>
<option value="c">Option C</option>
</tonic-select>
API
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Select box with id attribute. |
|
required |
boolean | Makes the select box required. | false |
disabled |
boolean | Makes the select box disabled. | false |
label |
string | Adds a label to the select box. | |
width |
string | Width of the select box. | 250px |
height |
string | Height of the select box. | |
radius |
string | Radius of the select box. | 2px |
multiple |
boolean | Show as multiple select. | |
size |
string | The number of visible items for a multiple select. | |
value |
string | The default value that will be selected. | |
tabindex |
number | Add a tabindex for the select box. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods
Method | Description |
---|---|
change() |
The native change event on the select input. |
loading(state) |
Adds loading to the current select box. |
Instance Members
Property | Description |
---|---|
option |
A getter/setter that provides the currently selected option from the select input inside the component. |
value |
A getter/setter that provides the current value of the select input from inside of the component. |
selectedIndex |
A getter/setter that provides the selected index of the select input inside the component. |
Split
A resizable Split
component that can contain any number of deeply nested
splits or child components (doesn't currently work on mobile).
Demo
Code
HTML
<tonic-split id="split-main" type="vertical">
<tonic-split-left width="40%">
<tonic-split id="split-secondary" type="horizontal">
<tonic-split-top height="60%">
<section>
Hello, World.
</section>
</tonic-split-top>
<tonic-split-bottom height="40%">
<section>
Hello, World.
</section>
</tonic-split-bottom>
</tonic-split>
</tonic-split-left>
<tonic-split-right width="60%">
<section>
Hello, World.
</section>
</tonic-split-right>
</tonic-split>
API
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Select box with id attribute. |
|
width |
string | Width of the select box. | 250px |
height |
string | Height of the select box. |
Instance Methods
Method | Description |
---|---|
toggle(panel, force) |
Show or hide an area of the split. For a vertical split, panel will be top or bottom . For a horizontal split, panel will be left or right . The boolean force parameter will force it to show (true) or hide (false). |
Instance Members
Property | Description |
---|---|
meta |
Gives you some information about the state of the split. |
Sprite
The Sprite
component produces an inline svg that contains symbols
which can be referred to by other components.
You should include the tonic-sprite
once, somewhere in the body, but before
other components that use it.
HTML
<tonic-sprite></tonic-sprite>
To use a symbol from another component, you can refer to the symbol by its id
property, for example...
JS
class MyComponent extends Tonic {
render () {
return this.html`
<tonic-icon symbol-id="close" size="40px"></tonic-icon>
`
}
}
Tabs
The tonic-tabs
and tonic-tab-panel
components create a tab list that activates sections when clicked on.
Demo
Code
The tabs are grouped under the tonic-tabs
component. Each tab should be a
tonic-tab
element, and is associated to a tonic-tab-panel
id with the
for
element.
The selected tab should specified by providing an id
as the value
for the selected
attribute on the tonic-tabs
component.
HTML
<tonic-tabs selected="tab-2" id="my-tabs">
<tonic-tab
id="tab-1"
for="tab-panel-1">
One
</tonic-tab>
<tonic-tab
id="tab-2"
for="tab-panel-2">
Two
</tonic-tab>
<tonic-tab
id="tab-3"
for="tab-panel-3">
Three
</tonic-tab>
</tonic-tabs>
HTML
<tonic-tab-panel id="tab-panel-1">
Content One
</tonic-tab-panel>
<tonic-tab-panel id="tab-panel-2">
Content Two
</tonic-tab-panel>
<tonic-tab-panel id="tab-panel-3">
Content Three
</tonic-tab-panel>
API
Properties
for tonic-tabs
Property | Type | Description | Default |
---|---|---|---|
selected |
string | Adds the id attribute. |
for tonic-tab
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. required |
|
for |
string | Adds the id attribute. required |
for tonic-tab-panel
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute. required |
Instance Methods & Members
for tonic-tabs
Method | Description |
---|---|
click() |
Click event. |
get value |
Get the currently selected tab. |
set selected(String) |
Set the currently selected tab. |
Events
for tonic-tabs
Event | Description |
---|---|
tabvisible |
Emitted when a tab becomes visible. Contains event.detail.id for which tab is visible. |
tabhidden |
Emitted when a tab becomes hidden. Contains event.detail.id for which tab is hidden. |
tabvisible , tabhidden |
Emitted when a tab is changed and get triggered both from click & keyboard events. |
Textarea
The Textarea
component creates a text area.
Demo
Code
Html
<tonic-textarea
placeholder="Placeholder"
rows="6"
id="my-textarea"
label="Text Area">
<!-- Content Goes Here -->
</tonic-textarea>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Text area with id attribute. required |
|
ariaLabelledby |
string | Sets the area-labelledby attribute. |
|
autofocus |
boolean | Enable autofocus on the text area. |
false |
cols |
string | Set number of columns. | |
disabled |
boolean | Text area with disabled attribute. |
false |
height |
string | Set height of text area. | 100% |
label |
string | Creates a label. | |
maxlength |
string | Set the maximum character length. | |
minlength |
string | Set the minimum character length. | |
name |
string | Text area with name attribute. |
|
persistSize |
boolean | Persist the resized width and height | false |
placeholder |
string | Add placeholder to text area. | |
radius |
string | Set radius of text area. | 2px |
readonly |
boolean | Set text area to readonly . |
false |
required |
boolean | Set text area to required . |
false |
resize |
string | Set to none to disable resize. |
|
rows |
string | Set number of rows. | |
spellcheck |
boolean | Enable spellcheck. | true |
tabindex |
number | Add a tabindex for the text area. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
width |
string | Set width of text area. |
Instance Methods & Members
Method | Description |
---|---|
value |
A getter/setter that provides the current value of the text area from inside of the component. |
ToasterInline
The ToasterInline
component creates an inline toaster item that appears on the
screen either for a duration or until the user acknowledges it.
Note: This component requires the
tonic-sprite
component.
Demo
Code
HTML
<tonic-toaster-inline id="toaster-1">
</tonic-toaster-inline>
JS
const toaster1 = document.getElementById('toaster-1')
const toasterLink1 = document.getElementById('toaster-link-1')
toasterLink1.addEventListener('click', e => {
toaster1.show()
})
An inline toaster that is displayed initially:
HTML
<tonic-toaster-inline
id="toaster-2"
dismiss="false"
display="true">
Displayed initially. Uses HTML.
</tonic-toaster-inline>
NOTE: An inline toaster item that is displayed initially must declare each property in the HTML and display it using display="true"
. This will create the notification.
Otherwise, the properties for the toaster item to be created must be specified in the javascript, where it is created.
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds an id attribute. |
|
name |
string | Adds a name attribute. |
|
title |
string | Adds a title. | |
message |
string | Adds a message. If no message attribute is provided the inner HTML will be used. | |
type |
string | Adds an alert type, success , warning , danger or info ). |
|
duration |
number | The duration that the component will be displayed before being hidden. | |
dismiss |
boolean | If set to false , the close button will not be added to the toaster item. |
|
display |
boolean | Specifies whether toaster is displayed initially. | false |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods & Members
Method | Description |
---|---|
show() |
Shows the toaster. |
hide() |
Hides a toaster item. |
click() |
Removes a toaster item. |
Toaster
The Toaster
component creates a container for all toaster items to be added to.
Note: This component requires the
tonic-sprite
component.
Demo
Code
The following code should be included once on the page:
HTML
<tonic-toaster id="tonic-toaster-example"></tonic-toaster>
To create a new toaster item:
JS
const notification = document.querySelector('tonic-toaster')
document
.getElementById('tonic-toaster-example')
.addEventListener('click', e => {
notification.create({
type: 'success',
title: 'Greetings',
message: 'Hello, World'
})
})
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds an id attribute. |
|
name |
string | Adds a name attribute. |
|
type |
string | Adds an alert type (success , warning , danger , info ). |
|
title |
string | Adds a title. | |
message |
string | Adds a message. | |
dismiss |
boolean | If set to false , the close button will not be added to the toaster. |
true |
duration |
number | Adds a duration. | center |
position |
string | (On Parent tonic-toaster Element) Position of the toaster items, can be left , right or center . |
center |
theme |
string | (On Parent tonic-toaster Element) Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods & Members
Method | Description |
---|---|
click() |
Removes a toaster item. |
create() |
Creates a toaster item. |
destroy() |
Removes a toaster item. |
Toggle
The Toggle
component creates a toggle.
Demo
Code
HTML
<tonic-toggle
id="toggle-example"
label="Change">
</tonic-toggle>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
id |
string | Adds the id attribute required |
|
name |
string | Adds the name attributes. |
|
disabled |
boolean | Makes the toggle disabled. | false |
checked |
boolean | Turns the toggle "on". | false |
label |
string | Adds a label to the toggle | |
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods
Method | Description |
---|---|
change() |
Bind to change event. |
Instance Members
Method | Description |
---|---|
value |
A getter/setter that returns the current value (state) of the toggle. |
Tooltip
The Tooltip
component creates a dynamically positioned pop-up tooltip filled with custom content that shows during the hover
state of the corresponding trigger element.
Demo
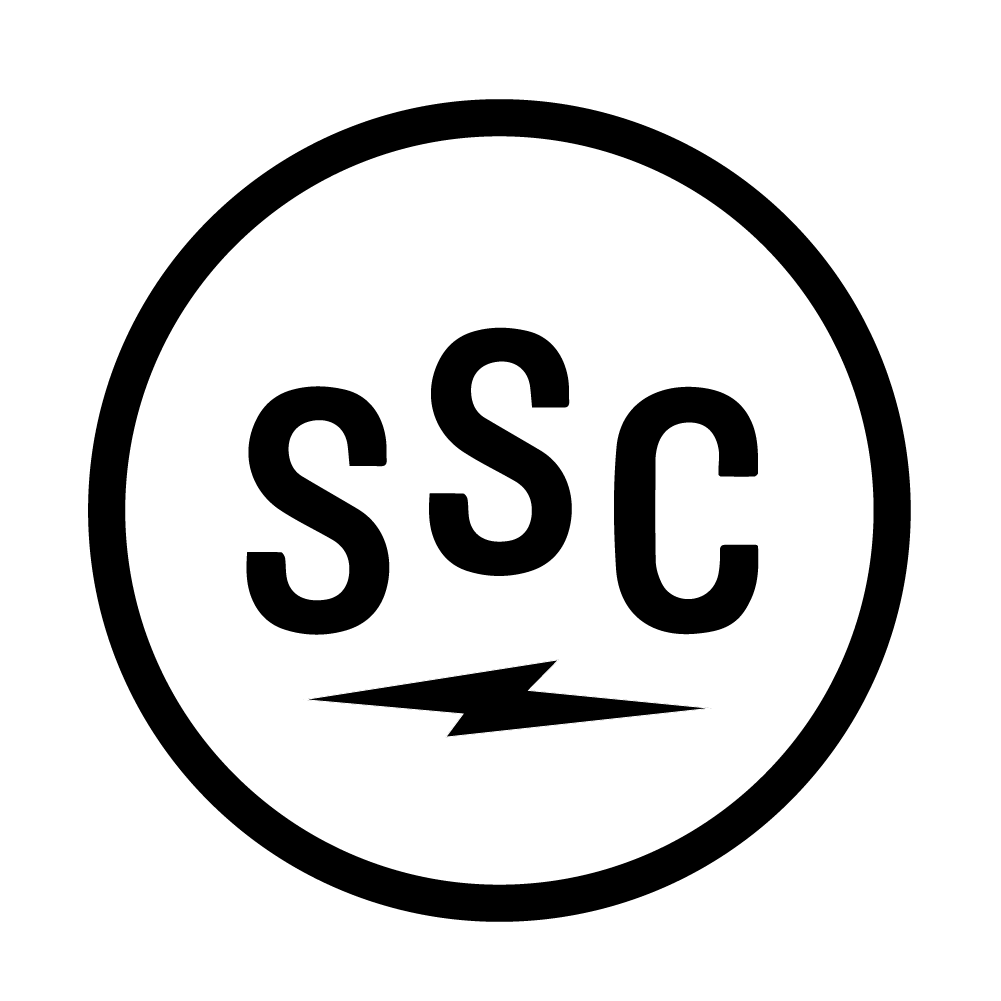
Code
The element that will be used to trigger the display of the tooltip must contain an id
that matches the for
attribute on the tonic-tooltip
element.
HTML
<span id="tonic-tooltip-example">Hover over this text</span>
<tonic-tooltip for="tonic-tooltip-example">
<img src="https://socketsupply.co/images/monogram.png" width="100px">
</tonic-tooltip>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
for |
string | Adds a for attribute. required |
|
width |
string | Changes the width style. |
|
height |
string | Changes height style. |
|
theme |
string | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
Instance Methods & Members
Method | Description |
---|---|
show() |
Shows the tooltip. |
hide() |
Hides the tooltip. |
Windowed
A base class used for creating a windowed component.
If you need to render large data sets (hundreds of thousands of rows for example), you can use a technique known as windowing
. This technique renders a subset of your data, while giving the user the impression that all the data
has been rendered.
Demo
This demo generates the data after you click the overlay. Generating 500000 rows of data can take a second or two.
Code
HTML
<example-windowed row-height=30>
</example-windowed>
Api
Properties
Property | Type | Description | Default |
---|---|---|---|
row-height |
Number | Sets the height of each row. required | 30 |
rows-page-page |
Number | The total number of rows per page to render. | 100 |
height |
String | Sets the height of the outer container. | inherit |
theme |
String | Adds a theme color (light , dark or whatever is defined in your base CSS. |
light |
debug |
Boolean | Add alternating page colors. | false |
Instance Methods
Method | Description |
---|---|
load(Array) |
Loads an array of data. |
loaded() |
Called after the load function has been called. |
getRows() |
Returns an array of all rows that are currently loaded. |
getRow(Number) |
Get a row of data (returns an awaitable promise). |
Instance Methods For Implementers
Method | Description |
---|---|
render() |
Render the component, calling super.render() will render the row container structure. |
renderEmptyState() |
If implemented, should return a structure that represents a state where there are no rows. |
renderLoadingState() |
If implemented, should return a structure that represents a state where has not yet completed. |